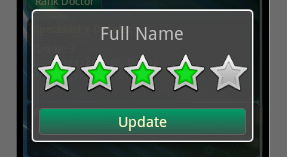
Activity Code:
Button rankBtn = (Button) findViewById(R.id.rank_button);
rankBtn.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
rankDialog = new Dialog(MyActivity.this, R.style.FullHeightDialog);
rankDialog.setContentView(R.layout.rank_dialog);
rankDialog.setCancelable(true);
ratingBar = (RatingBar)rankDialog.findViewById(R.id.dialog_ratingbar);
ratingBar.setRating(userRankValue);
TextView text = (TextView) rankDialog.findViewById(R.id.rank_dialog_text1);
text.setText(name);
Button updateButton = (Button) rankDialog.findViewById(R.id.rank_dialog_button);
updateButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
rankDialog.dismiss();
}
});
//now that the dialog is set up, it's time to show it
rankDialog.show();
}
});
layout/rank_dialog.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="2dip">
<TextView android:text="Doctor's Name" android:id="@+id/rank_dialog_text1"
android:textSize="24dp" android:layout_marginTop="10dp"
android:layout_width="wrap_content" android:layout_height="wrap_content"
android:layout_gravity="center"/>
<RatingBar
android:layout_marginTop="10dp" android:layout_marginBottom="10dp"
android:layout_width="wrap_content" android:layout_height="wrap_content"
android:clickable="true"
android:id="@+id/dialog_ratingbar" android:layout_gravity="center"
android:numStars="5" android:stepSize="1.0" android:rating="2"
android:isIndicator="false"/>
<Button android:id="@+id/rank_dialog_button"
android:layout_height="wrap_content"
android:text="Update" android:focusable="false"
android:layout_margin="5dip" android:textColor="#FFF8C6"
android:textSize="20dip" android:layout_width="fill_parent" />
</LinearLayout>
values/my_style.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="FullHeightDialog" parent="android:style/Theme.Dialog">
<item name="android:windowNoTitle">true</item>
</style>
</resources>